A few months ago I created a post which described a method for adding single signon services to an asp.net mvc website using Windows Azure ACS. The workflow involved allowing users to signup using forms authentication then associating their on-site identities with their facboook, google, yahoo and windows live identities. This post is meant to be a more detailed followup which will take you through some other things not covered in my original post.
The tutorial steps are as follows:
- Create an asp.net mvc 3 web application with forms authentication
- Register a new user
- Add Azure ACS sts reference to application
- Alter web.config to allow both sts and forms authentication
- Add database table to store mappings between identities and user accounts
- Create a custom class for extracting required claims
- Create a special controller action which Azure ACS will call to sign users in
- Create Hybrid login page with new functions
- Login with forms
- Login with ACS
- Prompt user to login and complete association process
Here is the workflow from the original post:
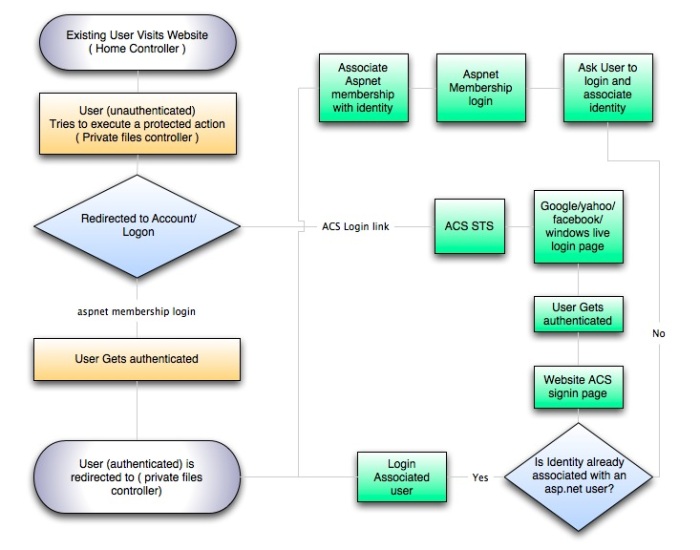
Assumptions about audience
Please ensure you have the following before proceeding with this tutorial
- Windows aure account
- Azure Access Control Services (ACS) working
- Windows Identity Framework Installed and working
- Basic understanding of Asp.net memberships
- Comfortable with Asp.net MVC
- Visual Studio 2010
- You have SQL express installed with required permissions
- You have experience with ACS/STS either through your own projects or tutorials such as on acs.codeplex.com
Again, If you have not been able to get ACS working using other samples and tutorials then it will be very difficult for you to follow this tutorial as I will not go into detailed explanations about setting up ACS, installing the Windows Identity Framework and Asp.net memberships.
Creating the Project
We will start by creating a new Asp.net MVC 3 project. I called mine MVC3MixedAuthenticationSample

Use the “Internet Application” template

Once the project is created add a reference to the Microsoft.IdentityModel assembly.
You should have a working Asp.net MVC 3 application at this point. The asp.net database will be created in the App_Data folder when we run the application.

Now run the project and register a new user. I called mine bob and gave him the password ‘password’.
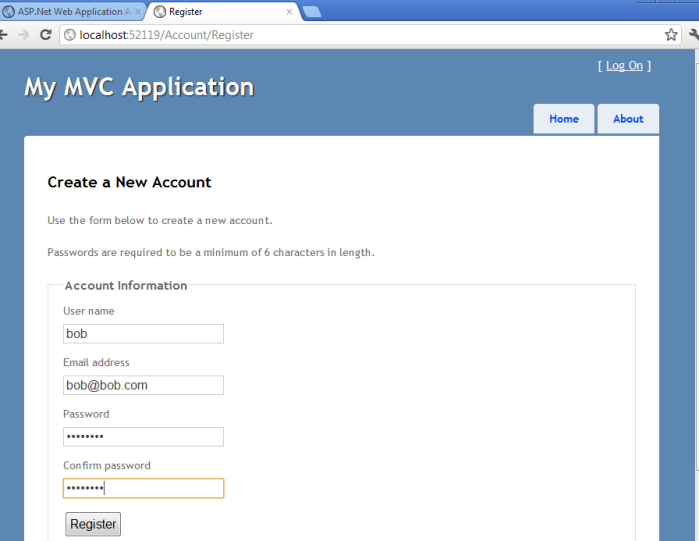
Your new user should now be logged into the site. You can also use the asp.net configuration tool to add a new user (you must build the project first). If you get any errors while attempting to add a new user you probably don’t have the correct permissions to the App_Data folder in the project or to sql express. There are several things you can try to resolve this issue that I can mention later if needed.
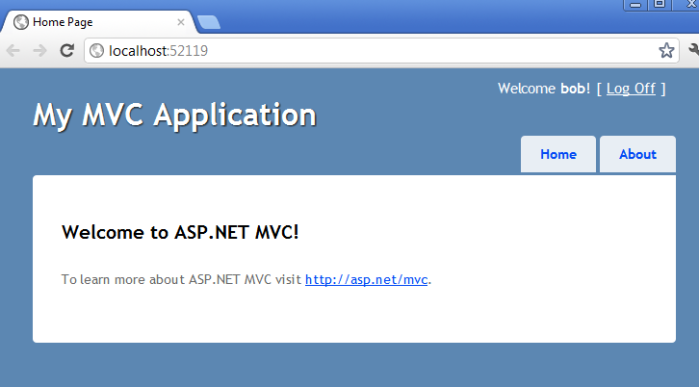
Our next step will be to include the newly created database in our project. This file is hidden by default so click the second icon from the left below “Solution Explorer” to show hidden files.
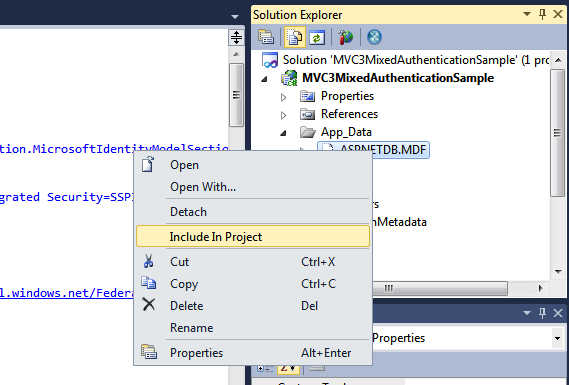
The database should now appear in the list of databases on the server explorer. Right click on the Tables node to add a new table.
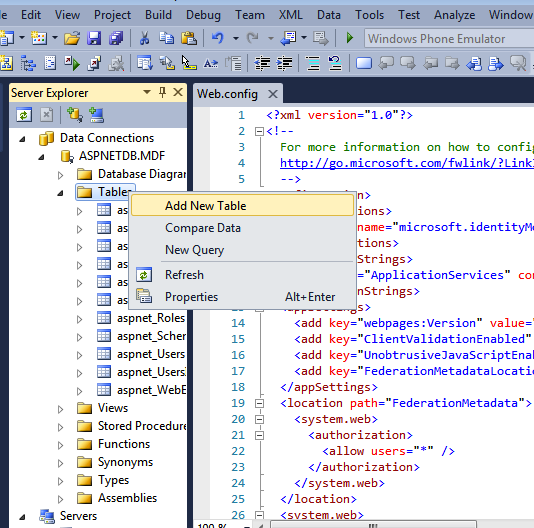
The new table will store the mappings between the acs identities and the local users.

Remember to enable auto increment for the primary key (IdentityID)
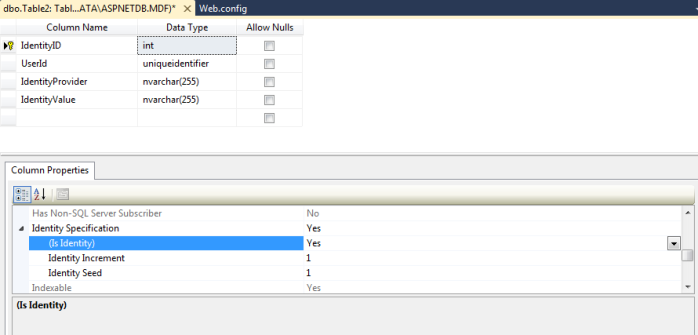
Save table and call it UserIdentity
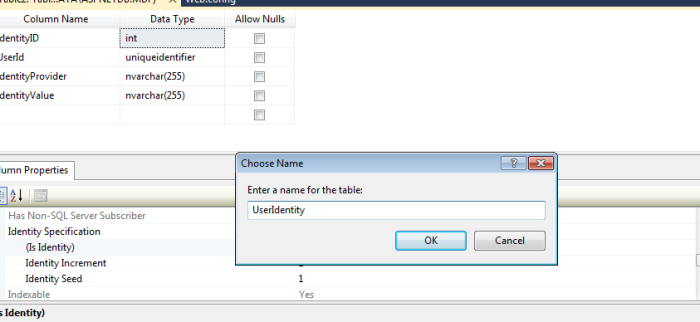
Now we need data access to our new table and any other tables related to it. I decided to use entity framework for this but you can use any ORM or simple ADO.NET if you like. Many people are reading this because they want to modify their existing projects so they may be using something else for data access. However, the principles are the same.
Create a new entity data model and opt to generate the entity from a database.
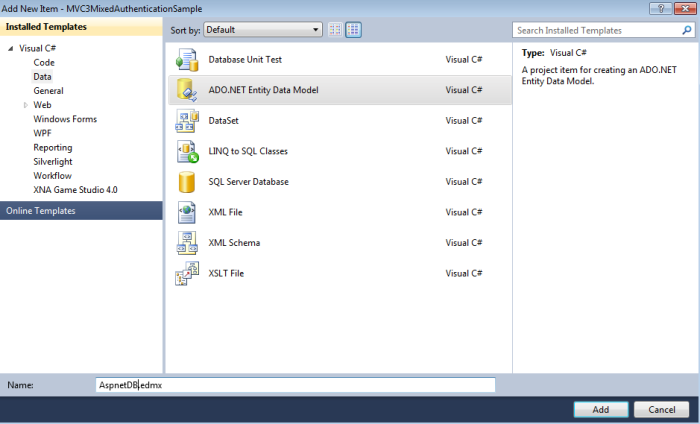
The “ApplicationServices” connection should already be selected. If not, select it. If it is not there, I would say add it but it not being there might be a symptom of a bigger issue.
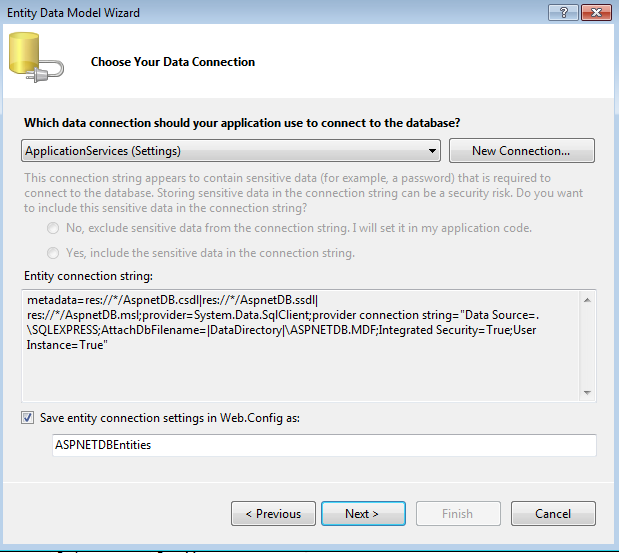
Add the UserIdentity table to the model. I have the aspnet_users table selected but it is not required since we will be using the asp.net membership api for everything related to local users.
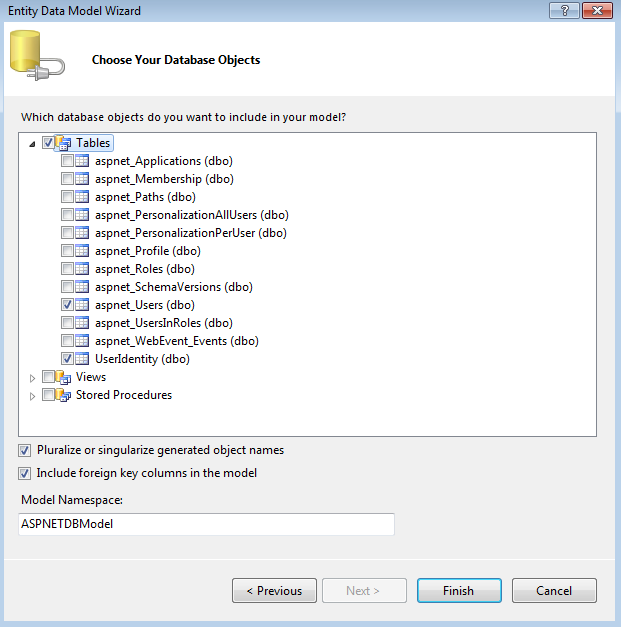
Now that the database and data access is setup we can add a reference to the Azure ACS STS.

Please fill in the url for the mvc application
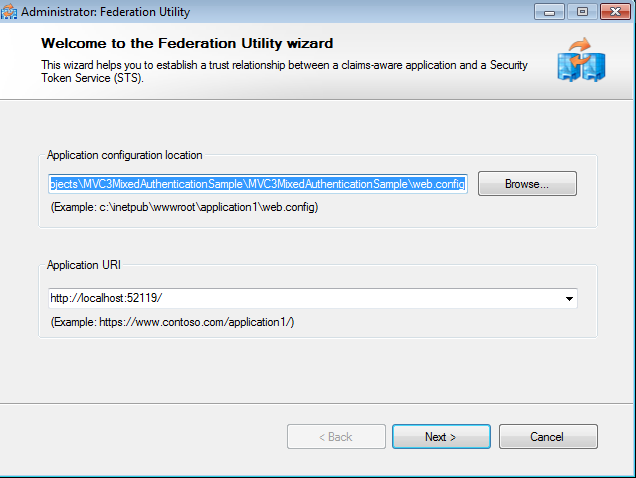
Select “Use an existing STS” and paste in the link to your ACS metadata. This can be found in your Azure control panel.

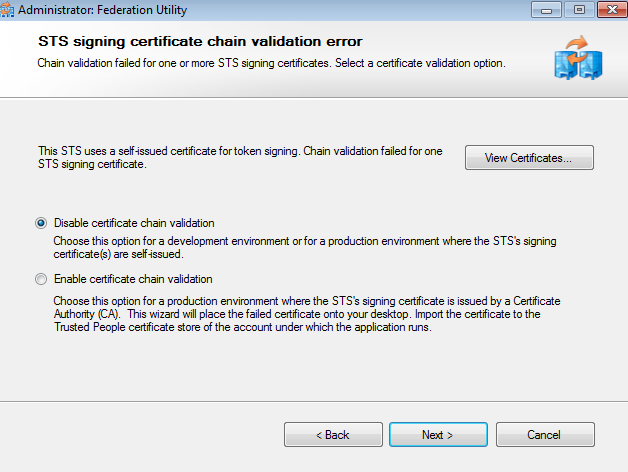
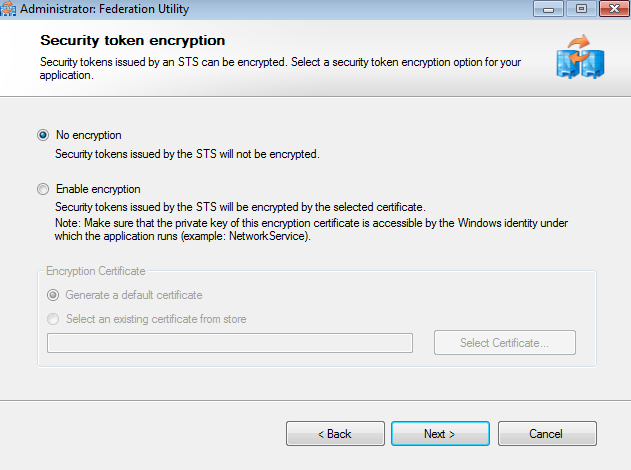
The following claims will be used to associate users with their identities


The wizard automatically disables forms authentication so the next step is to edit our web.config and turn forms authentication back on.
Open the web.config and find the attribute passiveRedirectEnabled and set it to false.
<wsFederation passiveRedirectEnabled="false" issuer="https://[namespace].accesscontrol.windows.net/v2/wsfederation" realm="http://localhost:52119/" requireHttps="false" />
Find the following and comment it out:
<authentication mode="None" />
Find the following and uncomment it:
<!--<authentication mode="Forms"><forms loginUrl="~/Account/LogOn" timeout="2880" /></authentication>-->
Find the following and comment it out:
<authorization>
<deny users="?" />
</authorization>
At this point you should be able to login to run the application and login with the user you created at the beginning.
Next we will Create a new class called IdentityClaim which we will use to extract the claims from the sts response. I added mine to the Models folder. Please note the using statement for Microsoft.IdentityModel.Claims.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using Microsoft.IdentityModel.Claims;
namespace MVC3MixedAuthenticationSample.Models
{
public class IdentityClaim
{
private string _identityProvider;
private string _identityValue;
public const string ACSProviderClaim = "http://schemas.microsoft.com/accesscontrolservice/2010/07/claims/identityprovider";
public IdentityClaim(IClaimsIdentity identity)
{
if (identity != null)
{
foreach (var claim in identity.Claims)
{
if (claim.ClaimType == ClaimTypes.NameIdentifier)
{
_identityValue = claim.Value;
}
if (claim.ClaimType == ACSProviderClaim)
{
_identityProvider = claim.Value;
}
}
}
}
public IdentityClaim()
{
_identityProvider = HttpContext.Current.Session["IdentityProvider"] as string;
_identityValue = HttpContext.Current.Session["IdentityValue"] as string;
}
public bool HasIdentity
{
get
{
return (!string.IsNullOrEmpty(_identityProvider) && (!string.IsNullOrEmpty(_identityValue)));
}
}
public string IdentityProvider
{
get
{
return _identityProvider;
}
}
public string IdentityValue
{
get
{
return _identityValue;
}
}
internal void SaveToSession()
{
HttpContext.Current.Session["IdentityProvider"] = _identityProvider;
HttpContext.Current.Session["IdentityValue"] = _identityValue;
}
public static void ClearSession()
{
HttpContext.Current.Session.Remove("IdentityProvider");
HttpContext.Current.Session.Remove("IdentityValue");
}
public static string ProviderNiceName(string identityProivder)
{
if (identityProivder.ToLower().Contains("windowslive"))
return "Windows Live";
if (identityProivder.ToLower().Contains("facebook"))
return "Facebook";
if (identityProivder.ToLower().Contains("yahoo"))
return "Yahoo";
if (identityProivder.ToLower().Contains("google"))
return "Google";
return identityProivder;
}
}
}
In my next post I will talk about creating the new controller action that will support Azure ACS signin and modifying the generated logOn page to include both the ACS buttons and the regular forms authentication option.